To convert a string to pandas datetime, you can use the pd.to_datetime() function from the pandas library. This function takes a string as input and converts it to a pandas datetime object. You can specify the format of the date string using the format parameter. If the date string is in a standard format like "YYYY-MM-DD", you can often skip specifying the format parameter. After converting the string to a pandas datetime object, you can perform various date-related operations and analyses on the data.
Best Python Books to Read in November 2024
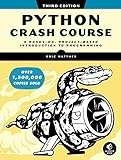
Rating is 4.8 out of 5
Python Crash Course, 3rd Edition: A Hands-On, Project-Based Introduction to Programming
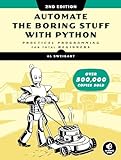
Rating is 4.7 out of 5
Automate the Boring Stuff with Python, 2nd Edition: Practical Programming for Total Beginners
- Language: english
- Book - automate the boring stuff with python, 2nd edition: practical programming for total beginners
- It is made up of premium quality material.
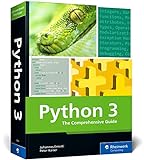
Rating is 4.6 out of 5
Python 3: The Comprehensive Guide to Hands-On Python Programming
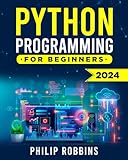
Rating is 4.5 out of 5
Python Programming for Beginners: The Complete Guide to Mastering Python in 7 Days with Hands-On Exercises – Top Secret Coding Tips to Get an Unfair Advantage and Land Your Dream Job!
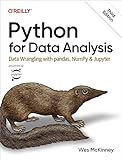
Rating is 4.4 out of 5
Python for Data Analysis: Data Wrangling with pandas, NumPy, and Jupyter
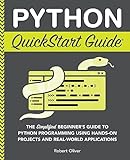
Rating is 4.2 out of 5
Python QuickStart Guide: The Simplified Beginner's Guide to Python Programming Using Hands-On Projects and Real-World Applications (QuickStart Guides™ - Technology)
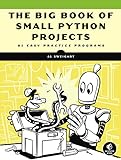
Rating is 4.1 out of 5
The Big Book of Small Python Projects: 81 Easy Practice Programs
What is the box parameter used in pd.to_datetime() function?
The box
parameter in the pd.to_datetime()
function is used to specify whether the output should be a single value or a structured NumPy array. When box=True
, the function returns a structured NumPy array with columns for year, month, day, etc. However, when box=False
(default), the function returns a single Pandas Timestamp object.
What are some common date formats for converting strings to pandas datetime?
- "%Y-%m-%d %H:%M:%S" - Year-month-day hour:minute:second
- "%m/%d/%Y %H:%M:%S" - Month/day/year hour:minute:second
- "%d-%m-%Y %H:%M:%S" - Day-month-year hour:minute:second
- "%Y/%m/%d %H:%M:%S" - Year/month/day hour:minute:second
- "%Y-%m-%d" - Year-month-day
- "%m/%d/%Y" - Month/day/year
- "%d-%m-%Y" - Day-month-year
- "%Y/%m/%d" - Year/month/day
What is the utc parameter used in pd.to_datetime() function?
The 'utc' parameter in the pd.to_datetime() function is used to specify whether the input datetime string is in Coordinated Universal Time (UTC) format or not. Setting utc=True tells the function to interpret the input as UTC time, while setting utc=False (the default) assumes the input is in local time.
How to convert a string to pandas datetime in UTC timezone?
You can convert a string to pandas datetime in UTC timezone by using the pd.to_datetime
function along with the tz_convert
method.
Here's an example:
1 2 3 4 5 6 7 8 9 10 |
import pandas as pd # Convert a string to pandas datetime date_str = '2022-10-15 12:30:00' date_utc = pd.to_datetime(date_str) # Convert pandas datetime to UTC timezone date_utc = date_utc.tz_localize('UTC') print(date_utc) |
This code will convert the input string 2022-10-15 12:30:00
to a pandas datetime in UTC timezone.
What is the unit parameter used in pd.to_datetime() function?
The unit parameter used in pd.to_datetime() function specifies the unit of the input data, such as 'ns' for nanoseconds, 'us' for microseconds, 'ms' for milliseconds, 's' for seconds, 'm' for minutes, 'H' for hours, 'D' for days, 'M' for months, 'Y' for years, etc. This parameter helps Pandas to correctly interpret the input data and convert it into datetime objects.