To convert an int to a string in p5.js, you can simply use the built-in JavaScript function toString()
. This function converts a number to a string and returns the result. You can call this function on an integer variable to convert it to a string data type. For example:
1 2 |
let myInt = 42; let myString = myInt.toString(); |
In this code snippet, myInt
is an integer variable with the value 42. By calling toString()
on myInt
, we convert it to a string and store the result in the myString
variable. You can now use myString
as a string in your p5.js sketch.
Best Javascript Books to Read in November 2024
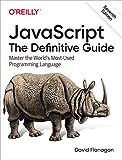
Rating is 5 out of 5
JavaScript: The Definitive Guide: Master the World's Most-Used Programming Language
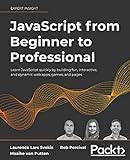
Rating is 4.9 out of 5
JavaScript from Beginner to Professional: Learn JavaScript quickly by building fun, interactive, and dynamic web apps, games, and pages
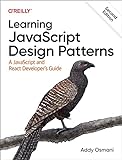
Rating is 4.8 out of 5
Learning JavaScript Design Patterns: A JavaScript and React Developer's Guide
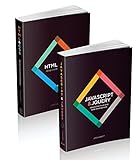
Rating is 4.7 out of 5
Web Design with HTML, CSS, JavaScript and jQuery Set
- Brand: Wiley
- Set of 2 Volumes
- A handy two-book set that uniquely combines related technologies Highly visual format and accessible language makes these books highly effective learning tools Perfect for beginning web designers and front-end developers
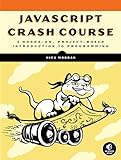
Rating is 4.6 out of 5
JavaScript Crash Course: A Hands-On, Project-Based Introduction to Programming
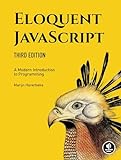
Rating is 4.4 out of 5
Eloquent JavaScript, 3rd Edition: A Modern Introduction to Programming
- It can be a gift option
- Comes with secure packaging
- It is made up of premium quality material.
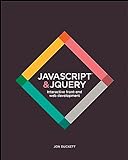
Rating is 4.3 out of 5
JavaScript and jQuery: Interactive Front-End Web Development
- JavaScript Jquery
- Introduces core programming concepts in JavaScript and jQuery
- Uses clear descriptions, inspiring examples, and easy-to-follow diagrams
How to convert a variable of type number to a string in p5.js?
In p5.js, you can convert a variable of type number to a string using the str()
function.
Here's an example:
1 2 3 4 |
let num = 42; let strNum = str(num); console.log(strNum); // Output: "42" |
In the example above, the str()
function converts the number variable num
to a string, which is then stored in the variable strNum
. The console.log()
function is used to print the string representation of the number.
How to convert an array of ints to an array of strings in p5.js?
In p5.js, you can easily convert an array of integers to an array of strings using the map()
function. Here is an example code snippet that demonstrates this:
1 2 3 4 |
let arrayInts = [1, 2, 3, 4, 5]; let arrayStrings = arrayInts.map(String); console.log(arrayStrings); |
In this code, we first define an array of integers arrayInts
. Then, we use the map()
function to apply the String
function to each element of the arrayInts
array, which converts each integer to a string. Finally, the resulting array of strings is stored in the arrayStrings
variable.
You can then access and use the array of strings arrayStrings
in your p5.js sketch as needed.
What is the significance of converting int to string in p5.js?
Converting an integer to a string in p5.js allows you to manipulate and display numeric values as text within your sketches. This can be useful for tasks such as displaying scores, labels, or user-inputted numbers on the screen. Additionally, converting an integer to a string can help you combine text and numbers in a single string, or use string manipulation functions to format the numeric value in a specific way. Ultimately, converting an int to a string in p5.js increases the flexibility and functionality of your code, allowing you to create more dynamic and interactive visual experiences.