To convert a list of strings to a map of strings with integer values in Kotlin, you can use the associateWith
function along with a transformation lambda.
Here's an example of how you can achieve this:
1 2 3 4 |
val list = listOf("apple", "banana", "orange") val map = list.associateWith { 0 } // Output: {apple=0, banana=0, orange=0} |
In this code snippet, we first create a list of strings containing "apple", "banana", and "orange". We then use the associateWith
function on the list to create a map where each string in the list is associated with an integer value of 0. The result is a map of strings with integer values.
Best Kotlin Books to Read in 2024
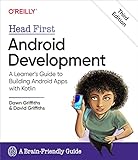
Rating is 4.9 out of 5
Head First Android Development: A Learner's Guide to Building Android Apps with Kotlin
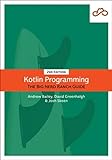
Rating is 4.8 out of 5
Kotlin Programming: The Big Nerd Ranch Guide (Big Nerd Ranch Guides)
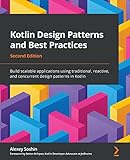
Rating is 4.6 out of 5
Kotlin Design Patterns and Best Practices: Build scalable applications using traditional, reactive, and concurrent design patterns in Kotlin, 2nd Edition
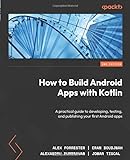
Rating is 4.3 out of 5
How to Build Android Apps with Kotlin: A practical guide to developing, testing, and publishing your first Android apps, 2nd Edition
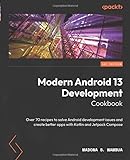
Rating is 4.2 out of 5
Modern Android 13 Development Cookbook: Over 70 recipes to solve Android development issues and create better apps with Kotlin and Jetpack Compose
What is the syntax for performing the conversion from List to Map with Kotlin?
In Kotlin, you can convert a list to a map using the associateBy
function.
Here is an example syntax:
1 2 3 4 |
val list = listOf("a", "b", "c") val map = list.associateBy({ it }, { it.length }) println(map) |
In this example, we have a list of strings and we are converting it to a map where the keys are the elements of the list and the values are the lengths of the strings.
How do I convert a list of strings to a map of strings to integers in Kotlin?
You can convert a list of strings to a map of strings to integers in Kotlin by using the associateWith
function in combination with the toMap()
function. Here's an example on how to do it:
1 2 3 4 5 |
val list = listOf("A" to "1", "B" to "2", "C" to "3") val map = list.associate { it } println(map) // Output: {A=1, B=2, C=3} |
In this example, we first create a list of pairs where the first element of the pair is the key and the second element is the value. Then we use the associate
function to convert the list to a map. Finally, we print the resulting map.
What is the simplest way to convert a list of strings to a map of strings to integers in Kotlin?
One simple way to convert a list of strings to a map of strings to integers in Kotlin is to use the associate
function along with a lambda expression. Here's an example:
1 2 3 4 |
val list = listOf("apple", "banana", "cherry") val map = list.associate { it to it.length } // Output: {apple=5, banana=6, cherry=6} |
In this example, the associate
function creates a new map where the keys are the elements of the list and the values are the length of each string.