To create a static array in Kotlin, you can use the arrayOf
function and assign it to a val
variable. This variable will hold the array with fixed values of a specific type. For example:
1
|
val staticArray = arrayOf(1, 2, 3, 4, 5)
|
In this example, staticArray
is a val
variable that holds a static array of integers with values 1, 2, 3, 4, and 5. You can access and modify the elements of the static array using index notation, such as staticArray[0]
to access the first element.
Static arrays allow you to define fixed values at compile-time, making it easier to work with constants or predefined values in your Kotlin code.
Best Kotlin Books to Read in 2024
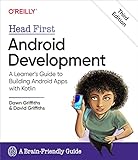
Rating is 4.9 out of 5
Head First Android Development: A Learner's Guide to Building Android Apps with Kotlin
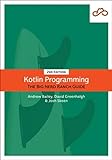
Rating is 4.8 out of 5
Kotlin Programming: The Big Nerd Ranch Guide (Big Nerd Ranch Guides)
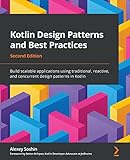
Rating is 4.6 out of 5
Kotlin Design Patterns and Best Practices: Build scalable applications using traditional, reactive, and concurrent design patterns in Kotlin, 2nd Edition
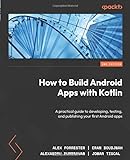
Rating is 4.3 out of 5
How to Build Android Apps with Kotlin: A practical guide to developing, testing, and publishing your first Android apps, 2nd Edition
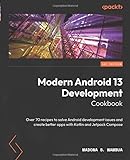
Rating is 4.2 out of 5
Modern Android 13 Development Cookbook: Over 70 recipes to solve Android development issues and create better apps with Kotlin and Jetpack Compose
How to initialize a static array in Kotlin?
In Kotlin, you can initialize a static array in two ways:
- Using the arrayOf function:
1
|
val numbers = arrayOf(1, 2, 3, 4, 5)
|
- Using the array constructor:
1
|
val numbers = intArrayOf(1, 2, 3, 4, 5)
|
Both of these methods will create an array of integers with the values 1, 2, 3, 4, and 5.
How to declare a fixed-size array in Kotlin?
In Kotlin, you can declare a fixed-size array using the arrayOf
function with fixed-size parameter or using the arrayOfNulls
function with fixed size parameter.
Here is an example of declaring a fixed-size array with specific values:
1
|
val numbers = arrayOf(1, 2, 3, 4, 5)
|
Here is an example of declaring a fixed-size array with null values:
1
|
val names = arrayOfNulls<String>(5)
|
In both examples, the arrays are fixed-size and cannot be resized once they are declared.
How to sort a static array in Kotlin?
To sort a static array in Kotlin, you can use the sortedArray()
function. Here is an example of how you can do this:
1 2 3 4 5 6 7 |
fun main() { val array = arrayOf(5, 2, 7, 3, 1) val sortedArray = array.sortedArray() println("Original array: ${array.joinToString()}") println("Sorted array: ${sortedArray.joinToString()}") } |
In this example, the sortedArray()
function is used to create a new sorted array from the original array. The joinToString()
function is then used to convert the arrays into strings for printing.
When you run this code, you should see the original array followed by the sorted array in the console.