To remove the first character from a Kotlin string, you can use the substring
function. You can specify the starting index as 1 to exclude the first character, like this:
1 2 |
val originalString = "example" val stringWithoutFirstChar = originalString.substring(1) |
In this example, stringWithoutFirstChar
will be equal to "xample"
, with the first character removed from the original string.
Best Kotlin Books to Read in 2024
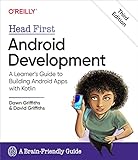
Rating is 4.9 out of 5
Head First Android Development: A Learner's Guide to Building Android Apps with Kotlin
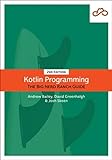
Rating is 4.8 out of 5
Kotlin Programming: The Big Nerd Ranch Guide (Big Nerd Ranch Guides)
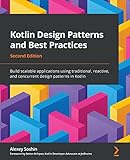
Rating is 4.6 out of 5
Kotlin Design Patterns and Best Practices: Build scalable applications using traditional, reactive, and concurrent design patterns in Kotlin, 2nd Edition
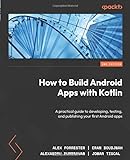
Rating is 4.3 out of 5
How to Build Android Apps with Kotlin: A practical guide to developing, testing, and publishing your first Android apps, 2nd Edition
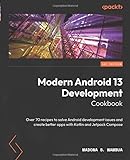
Rating is 4.2 out of 5
Modern Android 13 Development Cookbook: Over 70 recipes to solve Android development issues and create better apps with Kotlin and Jetpack Compose
How do you remove the starting character in a Kotlin string?
You can remove the starting character in a Kotlin string by using the substring()
function. Here is an example of how you can do this:
1 2 3 4 5 |
val str = "Hello" if (str.isNotEmpty()) { val newStr = str.substring(1) println(newStr) // Output will be "ello" } |
In this example, substring(1)
is used to remove the first character of the string "Hello" and the resulting string "ello" is printed to the console.
What is the best way to eliminate the initial character in a Kotlin string?
One way to eliminate the initial character in a Kotlin string is to use the substring
function. Here's an example code snippet demonstrating how to do this:
1 2 3 4 5 6 |
fun main() { val originalString = "example" val newString = originalString.substring(1) println(newString) } |
In this code snippet, the substring
function is called on the original string starting from index 1
, which skips the initial character in the string. The resulting newString
will have the initial character eliminated.
How to drop the first character from a Kotlin string without changing the original?
You can drop the first character from a Kotlin string without changing the original string by using the substring
function. Here's an example:
1 2 3 4 5 6 7 |
fun main() { val originalString = "hello" val newString = originalString.substring(1) println("Original String: $originalString") println("New String: $newString") } |
Output:
1 2 |
Original String: hello New String: ello |
In this example, the substring(1)
function is used to create a new string by skipping the first character of the original string. The original string remains unchanged.