To rename a category name in discord.js, you can use the setName() method on the CategoryChannel object. First, you need to fetch the category channel using its ID or name. Once you have the category channel object, you can call the setName() method with the new name you want to assign to the category. Make sure to handle any errors that may occur during the renaming process.
Best Javascript Books to Read in November 2024
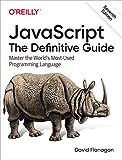
Rating is 5 out of 5
JavaScript: The Definitive Guide: Master the World's Most-Used Programming Language
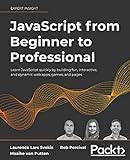
Rating is 4.9 out of 5
JavaScript from Beginner to Professional: Learn JavaScript quickly by building fun, interactive, and dynamic web apps, games, and pages
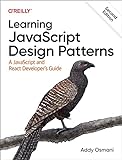
Rating is 4.8 out of 5
Learning JavaScript Design Patterns: A JavaScript and React Developer's Guide
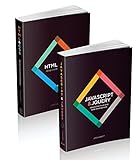
Rating is 4.7 out of 5
Web Design with HTML, CSS, JavaScript and jQuery Set
- Brand: Wiley
- Set of 2 Volumes
- A handy two-book set that uniquely combines related technologies Highly visual format and accessible language makes these books highly effective learning tools Perfect for beginning web designers and front-end developers
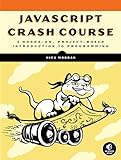
Rating is 4.6 out of 5
JavaScript Crash Course: A Hands-On, Project-Based Introduction to Programming
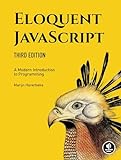
Rating is 4.4 out of 5
Eloquent JavaScript, 3rd Edition: A Modern Introduction to Programming
- It can be a gift option
- Comes with secure packaging
- It is made up of premium quality material.
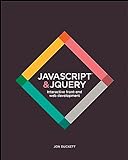
Rating is 4.3 out of 5
JavaScript and jQuery: Interactive Front-End Web Development
- JavaScript Jquery
- Introduces core programming concepts in JavaScript and jQuery
- Uses clear descriptions, inspiring examples, and easy-to-follow diagrams
How do you change the name of a category in discord.js?
To change the name of a category in discord.js, you can use the following code:
1 2 3 4 5 6 7 |
// Find the category by ID const category = message.guild.channels.cache.get('category_id'); // Change the name of the category category.setName('new_category_name') .then(updatedCategory => console.log(`Updated category name to ${updatedCategory.name}`)) .catch(console.error); |
Replace 'category_id'
with the ID of the category you want to change the name of, and 'new_category_name'
with the new name you want to set for the category.
What function allows you to change the name of a category in discord.js?
The function that allows you to change the name of a category in discord.js is setName()
. This function is used to change the name of a particular category to a new name specified by the user.
How to edit a category name in discord.js?
To edit a category name in Discord.js, you can use the setName()
method on the CategoryChannel object. Here is an example code snippet on how to edit a category name:
1 2 3 4 5 6 7 8 9 |
// Assume category is a reference to the CategoryChannel object you want to edit category.setName("new category name") .then(updatedCategory => { console.log(`Category name updated to: ${updatedCategory.name}`); }) .catch(error => { console.error(`Error updating category name: ${error}`); }); |
In this code snippet, we first call the setName()
method on the category
object with the new name as a parameter. This method returns a Promise that resolves to the updated CategoryChannel object. We can then log the updated category name to the console. If there is an error updating the category name, we catch the error and log it to the console.