To display a tkinter window in Linux, you need to first install tkinter if it is not already present on your system. You can do this by running the command "sudo apt-get install python3-tk" in your terminal.
Once tkinter is installed, you can create a tkinter window by writing a Python script that imports the tkinter module and defines a window with the tk.Tk() method. You can then add widgets to the window and configure them as needed.
To run the script and display the tkinter window in Linux, simply navigate to the location of your script in the terminal and run the command "python3 script_name.py" (replace "script_name.py" with the name of your Python script).
The tkinter window should then appear on your screen in Linux, allowing you to interact with the widgets and contents you have added to it.
Best Python Books to Read in June 2025
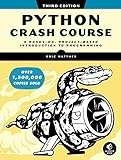
Rating is 4.8 out of 5
Python Crash Course, 3rd Edition: A Hands-On, Project-Based Introduction to Programming
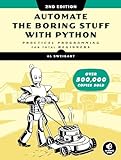
Rating is 4.7 out of 5
Automate the Boring Stuff with Python, 2nd Edition: Practical Programming for Total Beginners
- Language: english
- Book - automate the boring stuff with python, 2nd edition: practical programming for total beginners
- It is made up of premium quality material.
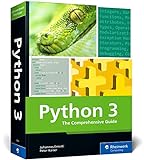
Rating is 4.6 out of 5
Python 3: The Comprehensive Guide to Hands-On Python Programming
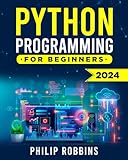
Rating is 4.5 out of 5
Python Programming for Beginners: The Complete Guide to Mastering Python in 7 Days with Hands-On Exercises – Top Secret Coding Tips to Get an Unfair Advantage and Land Your Dream Job!
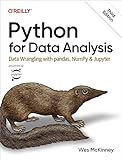
Rating is 4.4 out of 5
Python for Data Analysis: Data Wrangling with pandas, NumPy, and Jupyter
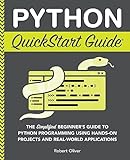
Rating is 4.2 out of 5
Python QuickStart Guide: The Simplified Beginner's Guide to Python Programming Using Hands-On Projects and Real-World Applications (QuickStart Guides™ - Technology)
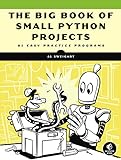
Rating is 4.1 out of 5
The Big Book of Small Python Projects: 81 Easy Practice Programs
How to close a tkinter window in Linux?
To close a tkinter window in Linux, you can simply click the close button (usually an "X" icon) at the top right corner of the window.
Alternatively, you can use the destroy()
method of the tkinter window object to close it programmatically. Here is an example:
1 2 3 4 5 6 7 8 9 10 |
import tkinter as tk # Create a tkinter window root = tk.Tk() # Display the window root.mainloop() # Close the window programmatically root.destroy() |
This will close the tkinter window when the destroy()
method is called.
What is a canvas in tkinter?
In tkinter, a canvas is a widget that allows for drawing and displaying graphics such as lines, shapes, text, and images. It provides a platform for creating interactive and visually appealing applications by allowing users to create and manipulate graphical elements on a blank canvas. The canvas widget can be used along with other widgets to create versatile and dynamic user interfaces in Python GUI applications.
What is a button in tkinter?
A button in Tkinter is a widget that allows the user to perform an action when it is clicked. It is typically used to trigger a specific event or function in a GUI application. Buttons in Tkinter can be customized with text, images, colors, and more. To create a button in Tkinter, you can use the Button class and specify the parent widget, text, command, and other optional attributes.