To import p5.js into TypeScript, you can start by downloading the p5.js library and saving it in your project directory. Next, create a new TypeScript file in which you want to use p5.js functionalities. In this file, import p5.js by adding the following line at the top:
import * as p5 from 'p5';
You can then create a new p5 instance and define setup and draw functions as you would in a regular p5.js sketch. Remember to compile your TypeScript code using a TypeScript compiler like tsc before running it in a browser.
Best Javascript Books to Read in March 2025
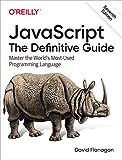
Rating is 5 out of 5
JavaScript: The Definitive Guide: Master the World's Most-Used Programming Language
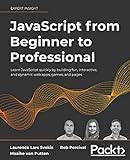
Rating is 4.9 out of 5
JavaScript from Beginner to Professional: Learn JavaScript quickly by building fun, interactive, and dynamic web apps, games, and pages
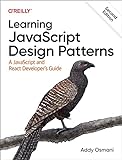
Rating is 4.8 out of 5
Learning JavaScript Design Patterns: A JavaScript and React Developer's Guide
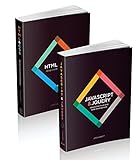
Rating is 4.7 out of 5
Web Design with HTML, CSS, JavaScript and jQuery Set
- Brand: Wiley
- Set of 2 Volumes
- A handy two-book set that uniquely combines related technologies Highly visual format and accessible language makes these books highly effective learning tools Perfect for beginning web designers and front-end developers
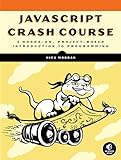
Rating is 4.6 out of 5
JavaScript Crash Course: A Hands-On, Project-Based Introduction to Programming
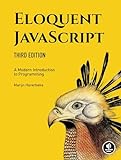
Rating is 4.4 out of 5
Eloquent JavaScript, 3rd Edition: A Modern Introduction to Programming
- It can be a gift option
- Comes with secure packaging
- It is made up of premium quality material.
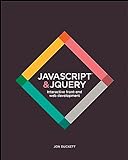
Rating is 4.3 out of 5
JavaScript and jQuery: Interactive Front-End Web Development
- JavaScript Jquery
- Introduces core programming concepts in JavaScript and jQuery
- Uses clear descriptions, inspiring examples, and easy-to-follow diagrams
What is the syntax to import p5.js into typescript?
To import p5.js into TypeScript, you can use the following syntax:
1
|
import * as p5 from 'p5';
|
This will import the entire p5.js library as a module named 'p5'. You can then use the p5 module to create sketches and interact with the p5.js API in your TypeScript code.
What is the recommended tool for managing p5.js dependencies in typescript projects?
The recommended tool for managing p5.js dependencies in TypeScript projects is npm or yarn. These package managers allow you to easily install and manage external libraries such as p5.js in your project. You can use npm or yarn to install the p5.js package and its typings, then import it into your TypeScript files as needed. Additionally, you can use a bundler like Webpack or Parcel to bundle your p5.js dependencies along with your TypeScript code for production.
How to make p5.js functions available in typescript files?
To make p5.js functions available in TypeScript files, you will need to install the p5.js library and typings for TypeScript.
Here are the steps to do so:
- Install the p5.js library using npm:
1
|
npm install p5
|
- Install p5.js typings for TypeScript using npm:
1
|
npm install @types/p5
|
- Set up your TypeScript file to import p5.js functions:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
import * as p5 from 'p5'; // Define a sketch using p5.js functions const sketch = (p: p5) => { p.setup = () => { p.createCanvas(400, 400); }; p.draw = () => { p.background(220); p.ellipse(200, 200, 50, 50); }; }; // Create a new p5 instance and pass the sketch function new p5(sketch); |
- Compile your TypeScript file using tsc:
1
|
tsc yourfile.ts
|
Now you should be able to use p5.js functions in your TypeScript file.