To read a CSV file into a pandas DataFrame, you first need to import the pandas library. Then, you can use the read_csv()
function from pandas to read the CSV file into a DataFrame. You can specify the file path of the CSV file as an argument to the read_csv()
function. Additionally, you can also specify other parameters such as delimiter, header, and column names while reading the CSV file. Once the CSV file is read into a DataFrame, you can perform various data manipulation and analysis tasks on the data using pandas methods and functions.
Best Python Books to Read in 2024
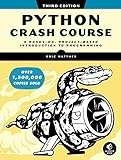
Rating is 4.8 out of 5
Python Crash Course, 3rd Edition: A Hands-On, Project-Based Introduction to Programming
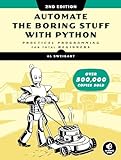
Rating is 4.7 out of 5
Automate the Boring Stuff with Python, 2nd Edition: Practical Programming for Total Beginners
- Language: english
- Book - automate the boring stuff with python, 2nd edition: practical programming for total beginners
- It is made up of premium quality material.
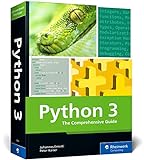
Rating is 4.6 out of 5
Python 3: The Comprehensive Guide to Hands-On Python Programming
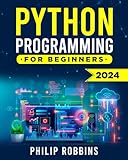
Rating is 4.5 out of 5
Python Programming for Beginners: The Complete Guide to Mastering Python in 7 Days with Hands-On Exercises – Top Secret Coding Tips to Get an Unfair Advantage and Land Your Dream Job!
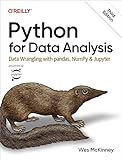
Rating is 4.4 out of 5
Python for Data Analysis: Data Wrangling with pandas, NumPy, and Jupyter
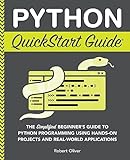
Rating is 4.2 out of 5
Python QuickStart Guide: The Simplified Beginner's Guide to Python Programming Using Hands-On Projects and Real-World Applications (QuickStart Guides™ - Technology)
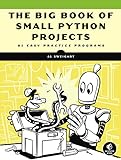
Rating is 4.1 out of 5
The Big Book of Small Python Projects: 81 Easy Practice Programs
What is the use of na_values in pandas read_csv function?
The na_values
parameter in the read_csv
function in pandas is used to specify a list of values that should be treated as missing values when reading a CSV file. This parameter allows you to define what values in the CSV file should be considered as NaN (Not a Number) or NULL values.
For example, if your CSV file contains certain strings or numbers that indicate missing data, you can specify those values in the na_values
parameter so that pandas will automatically recognize them as missing values. This can help in properly handling and cleaning the data during the data loading process.
How to skip specific rows while reading a CSV file in pandas?
You can skip specific rows while reading a CSV file in pandas by using the skiprows
parameter in the read_csv()
function.
For example, if you want to skip the first 2 rows of a CSV file, you can do so with the following code:
1 2 3 4 5 6 |
import pandas as pd # Read CSV file, skipping the first 2 rows df = pd.read_csv('file.csv', skiprows=[0,1]) print(df) |
In the code above, the skiprows
parameter is set to a list containing the row numbers that you want to skip. The row numbers start from 0, so skiprows=[0,1]
will skip the first 2 rows of the CSV file.
You can also specify a range of rows to skip by using a tuple, for example skiprows=(0,2)
will skip the first and third rows.
What is the syntax for reading a CSV file in pandas?
To read a CSV file in pandas, you can use the read_csv()
function. The syntax is as follows:
1 2 3 |
import pandas as pd data = pd.read_csv('filename.csv') |
In this syntax, replace 'filename.csv'
with the path to your CSV file. This function will read the CSV file and store the data in a DataFrame object named data
.