In Vite, in order to use namespaces, you can simply define the namespace in the component options object like this:
1 2 3 4 5 6 7 8 9 |
export default { namespace: 'myNamespace', data() { return { // Data properties }; }, // Other component options }; |
This will assign the specified namespace to the component. You can then access the namespace using this.$options.namespace
within the component's methods, computed properties, or lifecycle hooks.
Using namespaces can be helpful for organizing and distinguishing components in a large application, especially when components need to communicate with each other or share data.
Best Javascript Books to Read in 2024
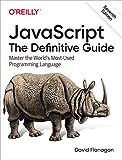
Rating is 5 out of 5
JavaScript: The Definitive Guide: Master the World's Most-Used Programming Language
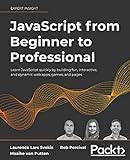
Rating is 4.9 out of 5
JavaScript from Beginner to Professional: Learn JavaScript quickly by building fun, interactive, and dynamic web apps, games, and pages
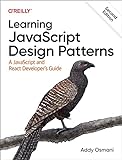
Rating is 4.8 out of 5
Learning JavaScript Design Patterns: A JavaScript and React Developer's Guide
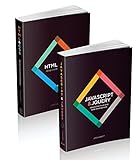
Rating is 4.7 out of 5
Web Design with HTML, CSS, JavaScript and jQuery Set
- Brand: Wiley
- Set of 2 Volumes
- A handy two-book set that uniquely combines related technologies Highly visual format and accessible language makes these books highly effective learning tools Perfect for beginning web designers and front-end developers
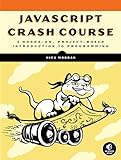
Rating is 4.6 out of 5
JavaScript Crash Course: A Hands-On, Project-Based Introduction to Programming
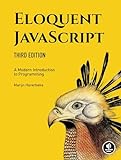
Rating is 4.4 out of 5
Eloquent JavaScript, 3rd Edition: A Modern Introduction to Programming
- It can be a gift option
- Comes with secure packaging
- It is made up of premium quality material.
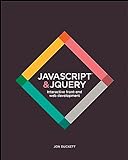
Rating is 4.3 out of 5
JavaScript and jQuery: Interactive Front-End Web Development
- JavaScript Jquery
- Introduces core programming concepts in JavaScript and jQuery
- Uses clear descriptions, inspiring examples, and easy-to-follow diagrams
What is the recommended naming convention for namespaces in Vite?
In Vite, the recommended naming convention for namespaces is to use PascalCase for consistency with JavaScript conventions. This means starting each word in the namespace with a capital letter, such as MyNamespace
. Using PascalCase helps to distinguish namespaces from variables, functions, and other types of identifiers in your code.
How to import a namespace in a Vite project?
To import a namespace in a Vite project, you can use the import * as <namespace> from '<module>'
syntax. Here's an example of how you can import a namespace in a Vite project:
- Create a new Vite project or open an existing one.
- In your JavaScript or TypeScript file, add the following import statement at the top:
1
|
import * as myNamespace from './myModule.js';
|
Replace myNamespace
with the desired namespace name and './myModule.js'
with the path to the module file that you want to import.
- You can now access the exported members of the namespace by using the namespace name followed by a dot, like this:
1 2 |
myNamespace.someFunction(); myNamespace.someVariable; |
Make sure that the module you are importing is properly exported with the export
keyword in the source file.
That's it! You have now successfully imported a namespace in your Vite project.
What is the syntax for defining a namespace in Vite?
In Vite, a namespace can be defined using the namespace
keyword followed by the desired namespace name. Here is an example:
1 2 3 4 |
// Define a namespace called 'myNamespace' namespace myNamespace { // Add your code here } |
You can then use this namespace to group related code and prevent naming conflicts with other parts of your application.